Building a CRUD Application with Laravel and MySQL: A Step-by-Step Guide
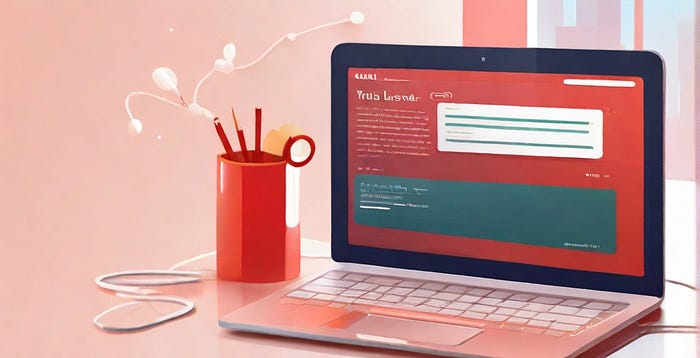
In the fast-paced world of web development, mastering CRUD operations (Create, Read, Update, Delete) is essential. These operations are the building blocks of most web applications, enabling dynamic content management and user interaction. This tutorial will guide you through creating a CRUD application using Laravel, a popular PHP framework, and MySQL, a widely-used database management system. Whether you’re new to Laravel or looking to refresh your skills, this guide will provide valuable insights and practical steps.
Step 1: Install Laravel
First, ensure Composer is installed on your system. Then, initiate a new Laravel project by executing:
composer create-project --prefer-dist laravel/laravel laravelMySQLExample
Step 2: Configure the MySQL Database
Modify the .env
file at the root of your Laravel project to set up your database connection. Ensure MySQL is installed and a database is ready for use. Update the following lines with your database name, username, and password:
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=your_database_name
DB_USERNAME=your_mysql_username
DB_PASSWORD=your_mysql_password
Step 3: Create a Migration for the “posts” Table
Generate a migration file for your “posts” table:
php artisan make:migration create_posts_table --create=posts
Edit the migration file in database/migrations
to define your table structure:
public function up()
{
Schema::create('posts', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->text('content');
$table->timestamps();
});
}
Execute the migration:
php artisan migrate
Step 4: Create a Model
Generate a Post model:
php artisan make:model Post
Laravel automatically links this model to your “posts” table.
Step 5: Create a Controller
Generate a controller with CRUD methods:
php artisan make:controller PostController
Implement methods for adding and retrieving posts in app/Http/Controllers/PostController.php
:
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\Post;
class PostController extends Controller
{
public function store(Request $request)
{
$post = new Post();
$post->title = $request->title;
$post->content = $request->content;
$post->save();
return response()->json(['message' => 'Post created successfully!']);
}
public function index()
{
$posts = Post::all();
return response()->json($posts);
}
}
Step 6: Define Routes
Configure routes in routes/web.php
:
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\PostController;
Route::get('/posts', [PostController::class, 'index']);
Route::post('/posts', [PostController::class, 'store']);
Step 7: Test Your Application
Launch the Laravel server:
php artisan serve
Test your application using Postman or similar tools by sending requests to your endpoints.
Extending the Laravel Example with CRUD Operations
Assuming you already have the setup from the previous example, let’s enhance our application by adding functionalities for reading a single post, updating a post, and deleting a post.
Reading a Single Post
To retrieve a specific post by its ID, we add a new method to our PostController
. This method utilizes the find
method provided by Eloquent to search for a post using its ID. If the post exists, it returns the post data; otherwise, it returns a "Post not found" message.
public function show($id)
{
$post = Post::find($id);
if ($post) {
return response()->json($post);
} else {
return response()->json(['message' => 'Post not found'], 404);
}
}
Updating a Post
To allow updates to an existing post, we introduce an update
method in the PostController
. This method finds the post by ID and updates its title
and content
with the new values provided in the request. It saves the updated post and returns a success message or a "Post not found" message if the post doesn't exist.
public function update(Request $request, $id)
{
$post = Post::find($id);
if ($post) {
$post->title = $request->title;
$post->content = $request->content;
$post->save();
return response()->json(['message' => 'Post updated successfully']);
} else {
return response()->json(['message' => 'Post not found'], 404);
}
}
Deleting a Post
The deletion of a post is handled by the destroy
method in the PostController
. This method attempts to find the post by its ID and then deletes it if found, returning a success message. If the post is not found, it returns an error message.
public function destroy($id)
{
$post = Post::find($id);
if ($post) {
$post->delete();
return response()->json(['message' => 'Post deleted successfully']);
} else {
return response()->json(['message' => 'Post not found'], 404);
}
}
Updating Routes for New Functionalities
With the new methods added to PostController
, we need to update our routes to include these functionalities. This involves defining routes for fetching a single post, updating a post, and deleting a post.
Route::get('/posts/{id}', [PostController::class, 'show']);
Route::put('/posts/{id}', [PostController::class, 'update']);
Route::delete('/posts/{id}', [PostController::class, 'destroy']);
Testing Your Application
You can now test these additional features by sending the appropriate HTTP requests:
- Read a Single Post: Send a GET request to
http://127.0.0.1:8000/posts/{id}
. - Update a Post: Send a PUT request to
http://127.0.0.1:8000/posts/{id}
withtitle
andcontent
in the request body. - Delete a Post: Send a DELETE request to
http://127.0.0.1:8000/posts/{id}
.